Image
Create visuals with prompts and use vision tools to describe or extract details from images.
<Image>
Server Component
A smart image component that either outputs text descriptions of an image, generates an image based on a prompt, or behaves just like a regular next/image
.
Describing an image
Live Example
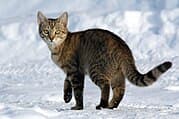
<Image
src="https://absolute-cat-url/tabbycat.jpg"
showResult // Optional - to render the alt-text visually
width={256}
height={170}
/>
Generating an image
Example
<Image
// The prompt is also used for the `alt` unless specified
prompt="A photograph of a cat wearing a cowboy hat"
model="dall-e-3"
size="1024x1024"
/>
Fallback To Standard next/image
<Image>
also works great even if you specify src
, alt
and no prompt
.
This is useful for cases where you might be dynamically setting props, and want to ensure that you'll always be able to fall back and display your images as expected.
See the Next.js <Image>
documentation for more information.
Live Example
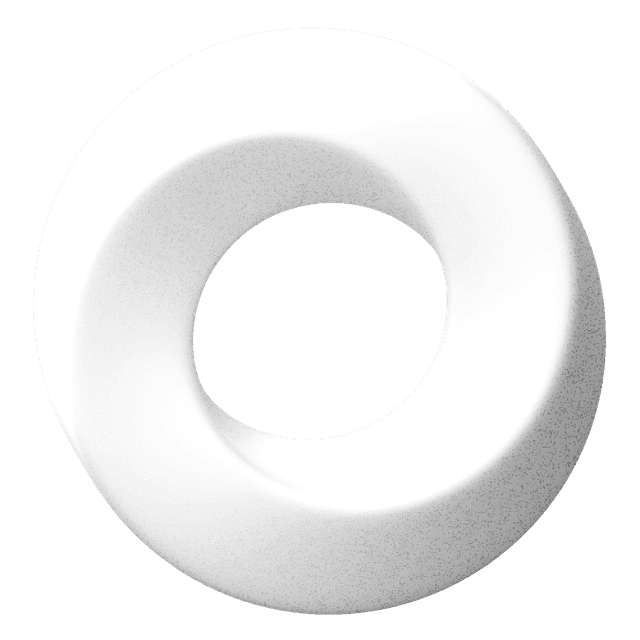
<Image
src="/images/MobiusStrip.png"
alt="A white coiling mobius strip"
width={300}
height={300}
/>
useImage
hook
A utility hook that allows for full access to the same features as Image
, in addition to being able to generate multiple images.
useImage
image description with src
'use client'
import { useImage } from '@trikinco/fullstack-components/client'
export default function Page() {
const { isLoading, isError, data } = useImage({
src: 'https://absolute-cat-url/tabbycat.jpg',
})
if (isLoading) {
return 'Describing image..."'
}
if (isError) {
return 'Could not describe image'
}
return <span>{data}</span>
}
useImage
image generation of multiple images with prompt
and n
'use client'
import { useImage } from '@trikinco/fullstack-components/client'
export default function Page() {
const { isLoading, isError, data } = useImage({
prompt: 'A friendly smiling robot',
n: 3,
})
if (isLoading) {
return 'Generating images...'
}
if (isError) {
return 'Could not generate images'
}
return data?.map((url) => (
<img
key={url}
src={url}
alt="A friendly smiling robot"
width="256"
height="256"
/>
))
}
Custom Server Component with getImage
import { getImage } from '@trikinco/fullstack-components'
export default async function Page() {
const { responseText } = await getImage({
prompt: `
An ethereal landscape in a far away fantasy land,
natural light,
golden hour
`,
})
return (
<img
src={responseText}
alt="An ethereal landscape in a far away fantasy land"
width="256"
height="256"
/>
)
}
Setup
Add image: handleImageRequest()
to the API route handler.
import {
handleFSComponents,
handleImageRequest,
type FSCOptions,
} from '@trikinco/fullstack-components'
const fscOptions: FSCOptions = {
image: handleImageRequest({
openAiApiKey: process.env.OPENAI_API_KEY || '',
}),
// Additional options and handlers...
}
const fscHandler = handleFSComponents(fscOptions)
export { fscHandler as GET, fscHandler as POST }
API Reference
Types
ImageOptions
Image API route handler options.Properties
openAiApiKeystring
- default`process.env.OPENAI_API_KEY`.
ImageRequestBodyinterface
Image generation or description request body. See OpenAI.ImageGenerateParams for full type information.Properties
srcstring
An absolute URL to the image to describe.- example'https://absolute-cat-url/tabbycat.jpg'
ImageGenerationResponse
The response body for `getImage`.Properties
responseTextT
The image generation response as an array or single base64 string or URL.creatednumber
The amount of image generations that have been performed by the API.dataarray
Represents the url or the content of an image generated by the OpenAI API.errorMessagestring
The error message if there was an error.ChatGptCompletionResponse
The response body for `getImage` and `getEnhancedImage` when describing an image.Properties
responseTextstring
The response text extracted from the completion message content.tokensUsednumber
Total number of tokens used in the request (prompt + completion).finishReasonstring
The reason the chat stopped. This will be `stop` if the model hit a natural stop point or a provided stop sequence, `length` if the maximum number of tokens specified in the request was reached, `content_filter` if content was omitted due to a flag from our content filters, `tool_calls` if the model called a tool, or `function_call` (deprecated) if the model called a function.errorMessagestring
The error message if there was an error.Components
ImagePropsImageGenerateProps | ImageDescribeProps | ImageProps as NextImageProps
Props to pass to the `<Image>` Server Component.ImageGeneratePropsinterface
Props specific to generating an image. Used in `ImageProps`.Properties
showResultboolean
Shows the image generation result base64 string or URL adjacent to the image.altstring
Alternative text describing the image, uses `prompt` if not provided.imageQualitystandard | hd
The quality of the image that will be generated. `hd` creates images with finer details and greater consistency across the image. This param is only supported for `dall-e-3`.imageStylenull | string
The style of the generated images. Must be one of `vivid` or `natural`. Vivid causes the model to lean towards generating hyper-real and dramatic images. Natural causes the model to produce more natural, less hyper-real looking images. This param is only supported for `dall-e-3`.onLoadfunction
Callback function invoked once the image is completely loaded and the `placeholder` has been removed.Parameters
eventSyntheticEvent | undefinedrequired
A React `HTMLImageElement` event object with no additional properties.responseImageResponse | undefined
The image generation response as a base64 string or URL.promptstring
A text description of the desired image. The maximum length is 1000 characters for `dall-e-2` and 4000 characters for `dall-e-3`.onErrorfunction
Callback function that is invoked if the image fails to load.Parameters
eventSyntheticEvent | undefinedrequired
A React `HTMLImageElement` event object with no additional properties.responseImageResponse | undefined
The image generation response as a base64 string or URL.promptstring
A text description of the desired image. The maximum length is 1000 characters for `dall-e-2` and 4000 characters for `dall-e-3`.ImageDescribePropsinterface
Props specific to describing an image. Used in `ImageProps`.Properties
srcstring
URL to the image to describe. Generates `alt` text. The `alt` text is then passed to the image and returned in `onLoad`.showResultboolean
Shows the image description text adjacent to the image.onLoadfunction
Callback function invoked once the image is completely loaded and the `placeholder` has been removed.Parameters
eventSyntheticEvent | undefinedrequired
A React `HTMLImageElement` event object with no additional properties.responseImageResponse | undefined
A text description response of the image.srcstring
An absolute URL to the image being described.- example'https://absolute-cat-url/tabbycat.jpg'
onErrorfunction
Callback function that is invoked if the image fails to load.Parameters
eventSyntheticEvent | undefinedrequired
A React `HTMLImageElement` event object with no additional properties.responseImageResponse | undefined
A text description response of the image.srcstring
An absolute URL to the image being described.- example'https://absolute-cat-url/tabbycat.jpg'
Imagefunction
import { Image } from '@trikinco/fullstack-components'
Parameters
propsImagePropsrequired
- linkImageProps
Hooks
useImagefunction
import { useImage } from '@trikinco/fullstack-components/client'
Parameters
bodyrequired
- linkImageRequestBody
configUseRequestConsumerConfig<ImageRequestBody>
Fetch utility hook request options without the `fetcher`. Allows for overriding the default `request` config.Returns
isLoadingboolean
Fetch loading state. `true` if the fetch is in progress.isErrorboolean
Fetch error state. `true` if an error occurred.errorunknown
Fetch error object if `isError` is `true`datastring | undefined
Fetch response data if the fetch was successful.- notereturns an array of strings if `n` is > 1.
refetchfunction
Refetches the data.Utilities
getImagefunction
import { getImage } from '@trikinco/fullstack-components'
Parameters
requestrequired
- linkImageRequestBody
optionsImageOptions
- linkImageOptions
getEnhancedImagefunction
import { getEnhancedImage } from '@trikinco/fullstack-components'
Parameters
propsImagePropsrequired
- linkImageProps
fetchImagefunction
import { fetchImage } from '@trikinco/fullstack-components/client'
Parameters
bodyrequired
- linkImageRequestBody
configRequestConfigOnly
Fetch utility request options without the `body`